Hello, everyone. Recently, I read that article about SVG Sprites and I wanted to share my experience working with both Image Sprites and SVG Sprites and how it can improve performance and simplify maintenance.
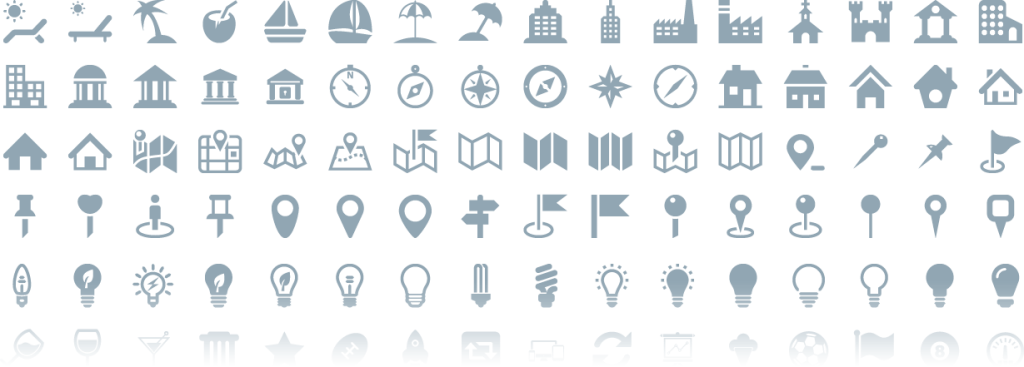
What Are Icon Sprites?
Imagine you have many small image icons for your website. Loading each one separately creates many HTTP requests and slows down the page. With icon sprites, you put all icons into one large image file (called a sprite sheet) and then use CSS to display only the section you need.
Why I Use Icon Sprites?
Here are some of the main benefits I get using Sprites:
- Less HTTP Requests: Instead of requesting each icon, the browser loads only one image file.
- Improved Performance: Fewer requests generally lead to faster page load times, which is good for both users and search engines.
- Easier Maintenance: When an icon needs updating, you change it in the sprite sheet and adjust the CSS accordingly.
How Icon Sprites Work
The idea is simple. You create one image that contains several icons, then use CSS properties like background-position
to show only the required part of the image. Let me explain the steps.
Step 1: Create the Sprite Sheet
Collect your icons and arrange them in one image file. It is important to leave some space between the icons to prevent accidental overlapping when you use CSS positioning.
You can use tools like Photoshop or online generators to create the sprite sheet. This process may seem a bit difficult at first, but it really pays off in performance.
Step 2: Write the CSS
Now we need to write CSS that tells the browser which part of the sprite sheet to show. This is done by setting the background image and using background-position
to shift the image inside the container.
Below is an example CSS:
/* Base style for all icons */
.icon {
display: inline-block;
width: 50px; /* Width of each icon */
height: 50px; /* Height of each icon */
background-image: url('sprite-sheet.png'); /* Path to the sprite sheet */
background-repeat: no-repeat;
}
/* Specific icon positions */
.icon-home {
background-position: 0 0; /* Shows the icon at top-left */
}
.icon-search {
background-position: -50px 0; /* Shifts the background left by 50px */
}
.icon-user {
background-position: -100px 0; /* Shifts further left */
}
Think of the background-position
values as coordinates. The first number controls the horizontal shift (negative moves left) and the second number controls the vertical shift.
Step 3: Add HTML Elements
After defining the CSS, you can now add the corresponding HTML elements:
<div class="icon icon-home"></div>
<div class="icon icon-search"></div>
<div class="icon icon-user"></div>
This simple structure displays the icons exactly where you want them on the page.
Advanced Techniques
Once you understand the basics, you can try some more advanced techniques to further improve your work.
Handling Retina Displays
For high-resolution screens, you can double the dimensions of your sprite sheet and scale it down with CSS. This ensures the icons look sharp on devices with retina displays.
.icon {
width: 50px;
height: 50px;
background-size: 200px 100px; /* Assuming the sprite sheet is twice as large */
}
Using SVG Sprites
Another modern approach is to use SVG sprites instead of raster images. SVGs are scalable, lightweight, and provide excellent quality on all devices. Here is an example of how to use SVG symbols:
<svg xmlns="http://www.w3.org/2000/svg" style="display: none;">
<symbol id="icon-home" viewBox="0 0 100 100">
<!-- Path for home icon -->
</symbol>
<symbol id="icon-search" viewBox="0 0 100 100">
<!-- Path for search icon -->
</symbol>
</svg>
<!-- Usage in HTML -->
<svg class="icon">
<use xlink:href="#icon-home"></use>
</svg>
<svg class="icon">
<use xlink:href="#icon-search"></use>
</svg>
This method is flexible and works very well with modern browsers.
A Real-World Example: Building a Navigation Bar
Let’s put these ideas into practice by building a simple navigation bar.
HTML Structure
<nav>
<a href="#" class="icon icon-home"></a>
<a href="#" class="icon icon-search"></a>
<a href="#" class="icon icon-user"></a>
</nav>
CSS Styling
nav a {
display: inline-block;
width: 50px;
height: 50px;
background-image: url('sprite-sheet.png');
background-repeat: no-repeat;
margin-right: 10px; /* Space between icons */
}
.icon-home {
background-position: 0 0;
}
.icon-search {
background-position: -50px 0;
}
.icon-user {
background-position: -100px 0;
}
This simple navigation bar uses icon sprites to display the icons efficiently and consistently.
Best Practices
Here are a few recommendations based on my experience:
- Optimize the Sprite Sheet: Use image compression to keep the file size small without losing quality.
- Group Related Icons: Organize your icons logically (e.g., navigation, social media) to simplify future updates.
- Test Across Devices: Always check that the icons display well on desktops, tablets, and mobile phones.
- Manage Caching: When updating the sprite sheet, use versioning in the URL (for example,
sprite-sheet.png?v=2
) to avoid caching problems.
Conclusion
Icon and SVG sprites in CSS are a very useful technique for any frontend developer looking to improve website performance. Combining multiple icons into a single image file reduces server requests and simplifies the process of updating icons.
Feel free to try this approach in your next project. If you have any questions or suggestions, do not hesitate to reach out 🙂 And See you in the next episode.
3 Comments
Worth noting if you create your icons as symbols in an Illustrator file and place each on a layer under a blank root layer they will export in a production-ready format
Looks nice but it does not work in Safari -_-
Very helpful